Table of Contents
ToggleSwift Data Types: Fundamental Building Blocks in Programming
Swift data types are fundamental building blocks of any Swift program, used to define and manipulate data in different ways. Swift provides various data types, including basic types like integers and floats, as well as more complex types like arrays and dictionaries.
In this post, we will cover the following data types in Swift:
- Integers
- Floats and Doubles
- Booleans
- Character
- Strings
We will explore each data type in detail, including their properties, behavior, and use cases, to help you understand how to work with them effectively in your Swift programs.
Integers
Integers are a type of data in Swift that represent whole numbers without any decimal points. They are used extensively in programming to represent quantities, counts, indices, and other numerical values.
Swift provides several types of integers with different sizes and ranges. The most common integer type used in Swift is Int, which is a signed integer that can represent values between -2^63 to 2^63-1 on 64-bit platforms, and -2^31 to 2^31-1 on 32-bit platforms.
Swift also provides several other integer types such as UInt (an unsigned integer), Int8, Int16, Int32, and Int64, which have different sizes and ranges. For example, Int8 can represent values between -128 to 127, while UInt can only represent non-negative integers.
The choice of integer type depends on the range of values you want to represent and the amount of memory you want to use. For instance, if you know that a variable will only hold non-negative integers, it’s more efficient to use UInt instead of Int, as UInt uses all of its bits to represent the value, while Int uses one bit for the sign.
Here’s an example of how to use integers in Swift:
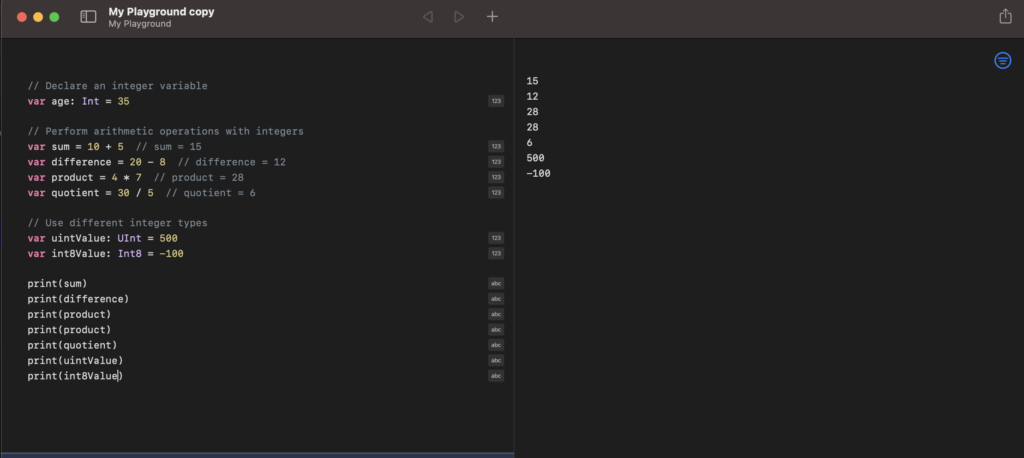
In this example, we declared an integer variable age and initialized it with the value 35. We also performed various arithmetic operations with integers and used different integer types such as UInt and Int8.
Floats and Doubles
Floating-point numbers are a type of data in Swift that represent decimal numbers, while integers represent whole numbers without decimal points. Floating-point numbers have a fractional part and an exponent, which allows them to represent a much wider range of values than integers.
Swift provides two floating-point number types: Float and Double. Float is a 32-bit floating-point number that can represent values with a precision of 6 decimal digits, while Double is a 64-bit floating-point number that can represent values with a precision of 15 decimal digits.
When to use each data type:
- Use integers for representing whole numbers, such as counts, indices, or any quantity that cannot be divided into smaller parts.
- Use floating-point numbers for representing values that can have fractional parts, such as measurements, coordinates, or any value that can be divided into smaller parts.
Here’s an example of how to use floats and doubles in Swift:
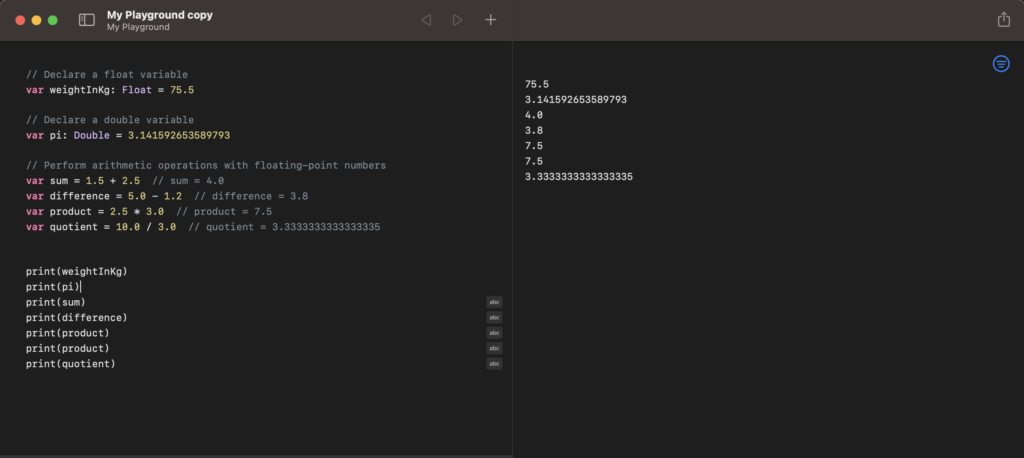
In this example, we declared a Float variable weightInKg and initialized it with the value 75.5, and a Double variable pi initialized with the value of pi. We also performed arithmetic operations with floating-point numbers, which resulted in values with fractional parts.
Booleans
A Boolean data type is a type of data in Swift that represents a logical value of either true or false. Booleans are commonly used in programming to represent conditions, comparisons, and decision-making.
In Swift, the Boolean data type is represented by the Bool type, which can only have two possible values: true and false. These values are used to represent the truth of a logical condition or statement.
Here’s an example of how to use Booleans in Swift:
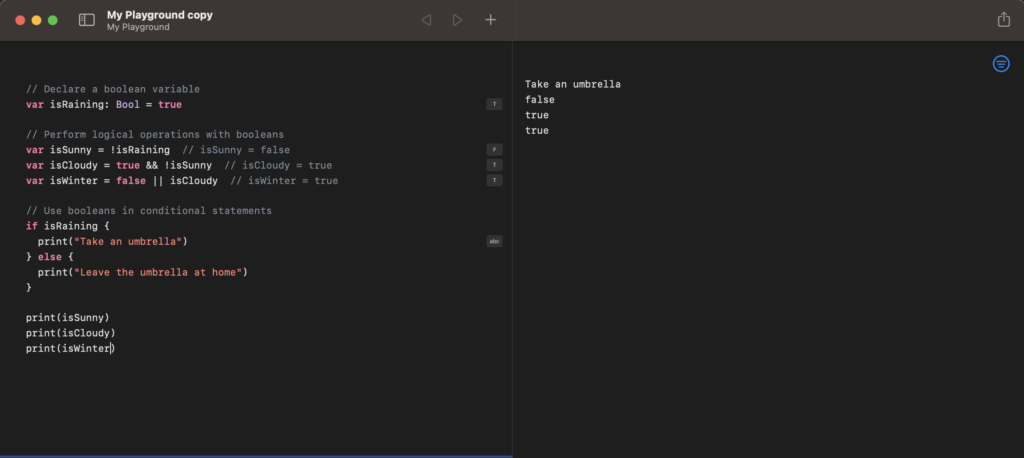
In this example, we declared a Boolean variable isRaining and initialized it with the value true. We also performed logical operations with Booleans using the logical negation (!), logical AND (&&), and logical OR (||) operators. These operations resulted in Boolean values that represent the truth of a logical statement.
We also used Booleans in conditional statements with an if statement, which printed a different message depending on the truth value of isRaining.
Character
A character data type is a type of data in Swift that represents a single character, such as a letter, number, or symbol. In Swift, character data is represented by the Character type.
Characters are commonly used in programming for text processing and manipulation. They can be used to represent individual letters, digits, or symbols in a string, or as standalone values for individual characters, such as for processing individual keystrokes in a user interface.
Here’s an example of how to use characters in Swift:
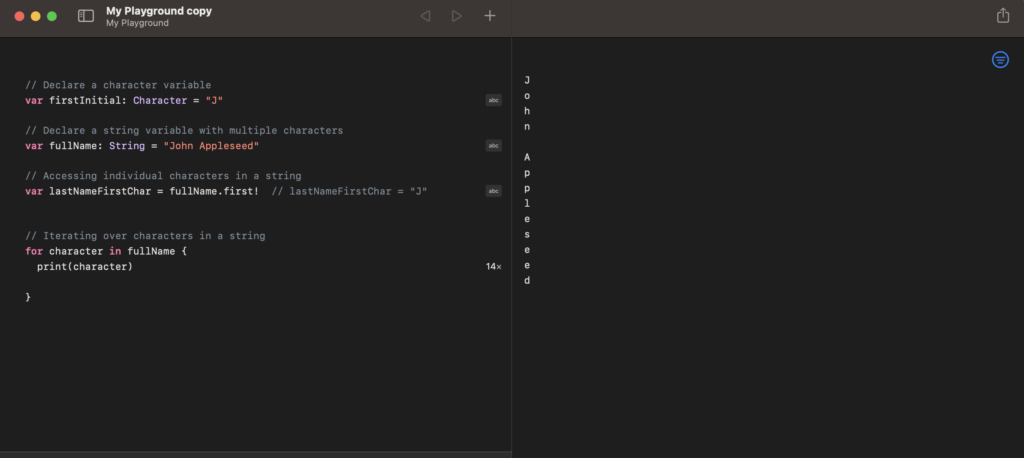
In this example, we declared a character variable firstInitial and initialized it with the value “J”. We also declared a string variable fullName with multiple characters.
We then accessed individual characters in a string using the first property of the string, which returned the first character in the string. We also demonstrated how to iterate over characters in a string using a for loop, which printed each character in the string to the console.
Note that characters in Swift are enclosed in single quotes (‘) and not double quotes (“), which are used for strings.
Strings
A string data type is a type of data in Swift that represents a sequence of characters. In Swift, string data is represented by the String type. Strings are commonly used in programming for text processing and manipulation.
Here are some common operations that can be performed on strings in Swift:
- Concatenation: joining two or more strings together to form a longer string.
var firstName = "John"
var lastName = "Appleseed"
var fullName = firstName + " " + lastName
print(fullName) // "John Appleseed"
In this example, we declared two string variables firstName and lastName, and concatenated them together using the + operator to form a new string fullName.
- Substring Extraction: A portion of a string can be extracted using the prefix and suffix methods:
var greeting = "Hello, world!"
var message = greeting.prefix(5) // "Hello"
var name = greeting.suffix(7) // "world!"
var middle = greeting.dropFirst(7).dropLast(7) // ", "
In this example, we declared a string variable greeting and extracted three different substrings from it using various methods:
- prefix(5) extracts the first five characters of the string.
- suffix(7) extracts the last seven characters of the string.
- dropFirst(7).dropLast(7) removes the first and last seven characters of the string, leaving only the middle portion.
- String interpolation: A way of inserting the value of a variable into a string:
let name = "Jane"
let age = 25
let message = "My name is \(name) and I am \(age) years old."
print(message) // "My name is Jane and I am 25 years old."
- Replacing substrings: A substring can be replaced with another string using the replacingOccurrences method:
let message = "Hello, world!"
let newMessage = message.replacingOccurrences(of: "Hello", with: "Hi")
print(newMessage) // "Hi, world!"
- Case conversion: Converting a string to uppercase or lowercase. This can be done using the uppercased and lowercased methods:
let myString = "Hello, world!"
let uppercaseString = myString.uppercased()
let lowercaseString = myString.lowercased()
- String length: Getting the number of characters in a string. This can be done using the count method:
let myString = "Hello, world!"
let length = myString.count
// length = 13
Strings are an essential part of programming in Swift, as they are used to store and manipulate text data. By understanding the different operations that can be performed on strings, you can write more sophisticated and powerful programs.
Conclusion
Congratulations! By reading this post, you’ve gained a solid understanding of the essential data types in Swift. With this knowledge, you’re now well-equipped to dive deeper into Swift programming and build exciting applications with confidence. These data types are the building blocks of any Swift program, and understanding how to work with them effectively is crucial for success. For further exploration and in-depth understanding, you can refer to the Swift documentation for comprehensive information. Additionally, to expand your knowledge on Swift variables and constants, check out our comprehensive beginner’s guide. So go ahead, unleash your creativity and start building! Happy Coding!