Table of Contents
ToggleIntroduction
Welcome to the beginner’s guide to Swift variables and constants. As one of the most fundamental concepts in programming, variables and constants are essential building blocks for any Swift application. Understanding how to use them effectively is key to developing efficient, maintainable code. In this blog post, we’ll take a deep dive into the world of variables and constants in Swift. We’ll explore their differences, uses, and best practices, along with examples to help you master these concepts. Whether you’re new to programming or an experienced developer looking to expand your skills, this guide is the perfect starting point for understanding variables and constants in Swift.
What Are Swift Variables and Constants?
Variables and constants are fundamental concepts in programming and are widely used in the Swift programming language.
In Swift:
A variable is a container that holds a value, which can be changed or modified during the execution of a program.
A constant is also a container that holds a value, but once assigned, the value cannot be changed during the program execution.
To declare a variable in Swift, you can use the “var” keyword, followed by the name of the variable, a colon, and the data type.
Variable Example:
var age: Int // declare a variable named age
age = 28 // update the value of the age variable to 28
In the example above, a variable named age is declared. The value of the variable is then updated to 28 using the assignment operator (=). If you re-assign this variable it will not result in a compiler error:
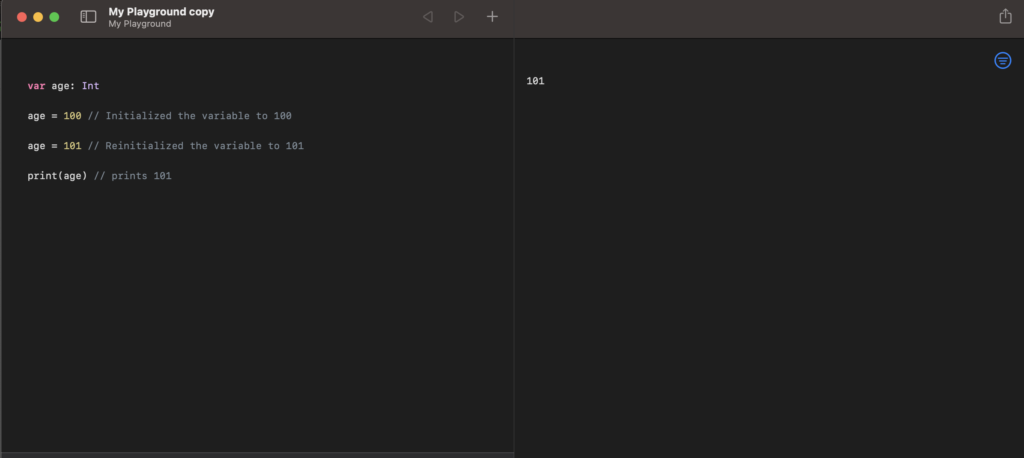
Constants Example:
let pi // declare a constant named pi
let message: String // declare a constant named message
In the example above, two constants are declared: pi and message. Since constants cannot be changed once they are assigned, if you were to give a value to these constants, and attempt to assign a new value to either of them, it would result in a compile-time error:
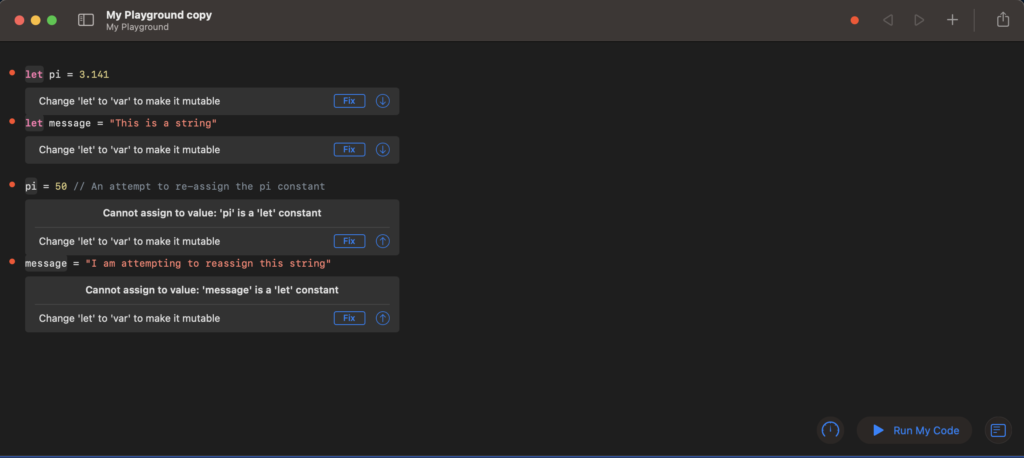
Why are Variables and Constants important?
Variables and constants are important in programming for a number of reasons:
- They allow programs to store and manipulate data. Without variables and constants, programs would have no way of storing information or keeping track of data.
- They enable programs to be flexible and adaptable. Variables allow programs to change and adjust their behavior based on changing conditions or user input.
- They help to make code more readable and maintainable. By using variables and constants to store values and data, programs become easier to read and modify, making it easier for developers to work on them.
- They enable programs to interact with the outside world. By storing values and data in variables and constants, programs can communicate with other programs or devices, read and write to files or databases, and perform other operations that require input or output.
Variables and constants are essential tools for building complex, powerful programs in Swift. Next, we are going to focus on declaring, and then initializing variables and constants.
What is Initialization?
We learned that variables are declared using the var keyword, and constants are declared using the let keyword. Now, let’s go deeper into what initialization is, and how to initialize variables and constants. When you initialize a variable or constant, you are assigning a value to it.
Declaring and initializing variables:
var age: Int // declare a variable named age of type Int
age = 27 // initialize the age variable with a value of 27
In the example above, a variable named age is declared using the var keyword and its data type is specified as Int. The variable is then initialized with a value of 27 using the assignment operator (=).
You can also declare and initialize a variable in a single line of code:
var age: Int = 27 // declare & initialize the age variable with a value of 27
In this example, the age variable is declared and initialized in one line using the var keyword, its data type (Int), and an initial value (27).
Declaring and initializing constants:
let pi = 3.14159 // declare a constant pi & initialize it with 3.14159
In the example above, a constant named pi is declared using the let keyword and initialized with a value of 3.14159. Since constants cannot be changed once they are assigned, there is no need to specify the data type explicitly in this case.
You can also declare and initialize a constant in a single line of code:
// declare and initialize a constant named message of type String
let message: String = "Hello, world!"
In this example, the message constant is declared and initialized in one line using the let keyword, its data type (String), and an initial value (“Hello, world!”)
By using the var and let keywords to declare and initialize variables and constants in Swift, you can easily store and manipulate data values in your code.
Understanding Type Inference and Type Annotation
What is Type Inference?
Type inference is a feature in Swift that allows the compiler to automatically deduce the data type of a variable or constant based on its initial value. This means that you can declare variables and constants without explicitly specifying their data type, and Swift will automatically assign the appropriate type based on the context in which they are used.
For example, if you declare a variable and initialize it with an integer value, Swift will automatically assign the integer data type to that variable:
var myNumber = 42
In this case, Swift infers that the type of myNumber is Int because the initial value is an integer.
Type inference can be used with most of the data types in Swift, including integers, floating-point numbers, strings, arrays, dictionaries, and more.
For example, you can use type inference to declare a constant and initialize it with a string:
let myMessage = "Hello, world!"
In this case, Swift infers that the type of myMessage is String because the initial value is a string.
Type inference can also be used with complex types, such as arrays and dictionaries. For example, you can declare an array of integers without specifying the data type explicitly:
let myNumbers = [1, 2, 3, 4, 5]
In this case, Swift infers that the type of myNumbers is [Int] because the initial value is an array of integers.
Using type inference can help make your code more concise and readable, as you don’t need to specify the data type explicitly when declaring variables and constants. It also reduces the risk of errors due to mismatched data types, as Swift ensures that the inferred data type is compatible with the initial value.
What is Type Annotation?
Type Annotation in Swift is a way to explicitly specify the data type of a variable or constant when it’s declared, instead of relying on the compiler to infer it automatically. This is done by adding a colon followed by the data type to the variable or constant declaration.
Here is an example of how to use Type Annotation in Swift:
// Declare an integer variable with type annotation
var myNumber: Int
myNumber = 42
// Declare a string constant with type annotation
let myString: String = "Hello, World!"
In the first example, the variable myNumber is declared as an integer using Type Annotation, without an initial value. Later on, the variable is assigned the value of 42. Since the data type of myNumber was explicitly specified as an integer, the compiler knows that it should only accept integer values for this variable.
In the second example, the constant myString is declared as a string using Type Annotation, and is also initialized with the string value “Hello, World!”. Since the data type is explicitly specified, the compiler knows that it should only allow string values for this constant.
Type Inference versus Type Annotation
We learned that type inference and type annotation are two ways of declaring the data type of a variable or constant in Swift. Let’s go over some general guidelines on when to use each one:
Use Type Inference:
- When the initial value of the variable or constant makes the data type clear
- When you want to make the code more concise and readable by not explicitly specifying the data type
- When you’re working with simple data types and the compiler can accurately infer the data type
Use Type Annotation:
- When you want to be explicit about the data type of a variable or constant, especially when working with complex data types
- When the initial value of the variable or constant doesn’t make the data type clear
- When you’re working on a project that requires high performance and you need to ensure that the compiler is optimizing the code correctly
- When you’re collaborating with other developers and want to make the code more readable by being explicit about the data types
It’s important to note that both type inference and type annotation have their own advantages and disadvantages, and the decision to use one over the other depends on the specific requirements of the project and personal coding style. In general, it’s recommended to use type inference when possible, and only use type annotation when it’s necessary for clarity or performance reasons.
Naming Conventions
Naming conventions for variables and constants in Swift are important for writing clean, readable, and maintainable code. Here are some guidelines for naming conventions in Swift:
- Use descriptive names: Variable and constant names should clearly indicate what they represent. For example, instead of using “x” or “y” for coordinates, use “latitude” and “longitude”. This makes the code more readable and easier to understand.
- Avoid abbreviations: While abbreviations can sometimes save time and space, they can also make the code more difficult to read and understand. Use full words instead of abbreviations whenever possible.
- Use camel case: Camel case is a convention where the first letter of the first word is lowercase and the first letter of each subsequent word is capitalized. For example, “firstName”, “lastName”, and “phoneNumber”.
- Avoid spaces and special characters: Variable and constant names should not contain spaces or special characters such as hyphens, underscores, or periods. Use camel case to separate words instead.
- Use uppercase for constants: Constants should be written in all uppercase letters, with words separated by underscores. For example, “MAXIMUM_LENGTH” or “DEFAULT_COLOR”.
- Use singular nouns for variables: Variable names should use singular nouns rather than plural nouns. For example, “car” instead of “cars”.
- Avoid starting names with a number: Names cannot begin with a number, as it is reserved for literal values.
Using descriptive, meaningful, and consistent names for variables and constants in Swift can also make the code more readable, easier to understand, and easier to maintain.
Mutability: Variables versus Constants
In Swift, variables and constants are used to store values of different data types. The main difference between variables and constants is that variables are mutable, meaning their values can be changed after they are declared, while constants are immutable, meaning their values cannot be changed after they are declared.
So, when should you use a variable and when should you use a constant? Here are some general guidelines:
- Use a variable when you need to store a value that may change during the execution of your program. For example, you might use a variable to keep track of the score in a game, or to store a value that is calculated based on other inputs.
- Use a constant when you need to store a value that should not change during the execution of your program. For example, you might use a constant to store a value that is known at compile-time, or to store a value that is a configuration setting for your program.
Using constants instead of variables has several advantages, including:
- Improved readability and maintainability of your code: Since constants cannot be changed, it’s easier to reason about the behavior of your code and to understand the intent of the programmer.
- Better performance: Constants can be optimized by the Swift compiler, which can result in faster execution of your program.
- Fewer bugs: Constants reduce the risk of bugs caused by accidentally changing the value of a variable that should not change.
What are Data Types?
In Swift, variables and constants can have different data types that define the kind of values they can store. Here are some of the most common data types used in Swift:
- Int: The Int data type is used to store integer values, which are whole numbers without decimal points. For example, 42 or -10 are integer values.
- Float: The Float data type is used to store single-precision floating-point values, which are numbers with decimal points and smaller range than Double. For example, 3.14 or -0.5 are float values.
- Double: The Double data type is used to store double-precision floating-point values, which are numbers with decimal points and larger range than Float. For example, 3.14159265359 or -0.0001 are double values.
- String: The String data type is used to store a sequence of characters or text, such as “Hello, World!” or “John”.
- Bool: The Bool data type is used to store boolean values, which can be either true or false.
To specify the data type when declaring a variable or constant in Swift, you can use the colon (:) followed by the name of the data type. For example:
var myInteger: Int = 42
let myString: String = "Hello, World!"
var myBool: Bool = true
In this example, myInteger is declared as an Int, myString is declared as a String, and myBool is declared as a Bool. The equal sign (=) is used to assign an initial value to the variable or constant. Note that Swift supports type inference, which means that you don’t always need to specify the data type explicitly. In many cases, the compiler can infer the data type based on the initial value assigned to the variable or constant. For example:
var myInteger = 42
let myString = "Hello, World!"
var myBool = true
In this example, the data types of myInteger, myString, and myBool are inferred by the compiler based on their initial values.
What Are Literal Values In Swift?
In Swift, literal values are values that are explicitly specified in the code and represent a specific data type. Literal values can be used to assign a value to a variable or constant, or to pass a value as an argument to a function or method.
There are several types of literal values in Swift, including:
- String literals: String literals are used to represent a sequence of characters surrounded by double quotes, such as “Hello, World!”.
- Integer literals: Integer literals are used to represent whole numbers, such as 42 or -10.
- Floating-point literals: Floating-point literals are used to represent decimal numbers, such as 3.14 or -0.5.
- Boolean literals: Boolean literals are used to represent true or false values, such as true or false.
- Nil literals: Nil literals are used to represent a lack of a value and are represented by the keyword nil.
- Array literals: Array literals are used to represent an ordered collection of values of the same type surrounded by square brackets, such as [1, 2, 3].
- Dictionary literals: Dictionary literals are used to represent an unordered collection of key-value pairs surrounded by square brackets, such as [“name”: “John”, “e”: 30]
Literal values in Swift are useful for initializing variables and constants with initial values, specifying arguments for functions and methods, and for representing data in a clear and concise way.
Literal Examples
let myString = "Hello, World!"
print(myString)
In this example, the literal value “Hello, World!” is assigned to the constant myString using a string literal. The print() function is then used to output the value of myString to the console, which would output “Hello, World!” when executed.
Another example using an integer literal:
let myNumber = 42
print(myNumber)
In this example, the integer literal value 42 is assigned to the constant myNumber. The print() function is then used to output the value of myNumber to the console, which would output 42 when executed.
The Importance of Scope
In Swift, the scope of a variable or constant refers to the region of the code where the variable or constant is visible and accessible. There are two main types of scopes in Swift:
- Global scope: A variable or constant defined in the global scope is visible and accessible throughout the entire program, from any function or block of code. Global variables and constants are typically declared at the top of a Swift file, outside of any functions.
Here’s an example of a global constant:
let gravity = 9.81 // Global Constant
struct Ball {
let mass: Double
let radius: Double
let density: Double
func calculateWeight() -> Double {
let volume = (4/3) * Double.pi * pow(radius, 3)
let weight = mass * gravity
return weight
}
}
In this case, gravity is accessible from any function or block of code in the program. In this example, it is accessible to the calculateWeight function inside the Ball struct.
- Local scope: A variable or constant defined in a function or block of code is only visible and accessible within that function or block of code. Local variables and constants are typically used for temporary storage or calculations within a function.
Here’s an example of a local variable:
func calculateSum() {
let x = 10
let y = 20
let sum = x + y
print("The sum is \(sum)")
}
In this case, x, y, and sum are only accessible within the calculateSum() function.
To define a variable or constant within a function or block of code, simply include the definition within the braces of the function or block. For example:
func myFunction() {
let x = 5
var y = 10
// other code here
}
In this case, x is a local constant and y is a local variable, both of which are only accessible within the myFunction() function.
Variables and constants can be defined in both the global and local scopes in Swift. Global variables and constants are visible and accessible throughout the entire program, while local variables and constants are only visible and accessible within the function or block of code where they are defined.
Best Practices
Here are some best practices for working with variables and constants in Swift:
- Use camelCase for variable names: In Swift, it is a common practice to use camelCase naming convention for variables and constants. This means that the first letter of the first word in the name should be lowercase and the first letter of each subsequent word should be uppercase. For example, myVariableName or myConstantName.
- Initialize variables and constants with default values: It is a good practice to initialize variables and constants with default values whenever possible. This helps to avoid unexpected behavior in your code and makes it easier to reason about the values of your variables and constants. For example, you can initialize a variable with a default value like this: var myVariable = 0.
- Use constants for values that do not change: Whenever possible, use constants for values that do not change throughout the execution of your program. This makes your code more readable and easier to understand because you don’t have to track the changes to the value. For example, you can use a constant to represent the value of pi: let pi = 3.14159265359.
- Use descriptive variable names: It is important to use descriptive variable names that make it clear what the variable represents. This helps to make your code more readable and easier to understand. For example, instead of using x and y as variable names, use width and height to represent the dimensions of a rectangle.
- Avoid using global variables: It is generally considered a bad practice to use global variables because they can be modified from anywhere in your code, making it difficult to track down bugs. Instead, use local variables and pass them as parameters to functions when needed.
- Use type annotations when necessary: While Swift’s type inference is powerful, there may be cases where you need to explicitly specify the type of a variable or constant. This is especially true for complex types or when working with third-party libraries.
These best practices help keep the code clean, readable, and maintainable.
Conclusion
Congratulations! By reading this post, you’ve gained a solid understanding of the essential data types in Swift. These data types are crucial building blocks for any Swift program. Understanding them is key to success in Swift programming. The blog covered important topics such as declaration, initialization, naming conventions, data types, mutability, scope, type inference, and best practices for Swift variables and constants. This foundational understanding will strengthen your Swift programming skills.
For further insights into Swift data types and to expand your knowledge, it’s recommended to explore our next blog on Swift data types.
For a deeper dive and comprehensive understanding, consult the Swift documentation. Mastery of variables and constants is pivotal for crafting efficient code in Swift. With consistent practice and dedication, you’ll enhance your programming prowess, enabling you to write more impactful and efficient code. Wishing you success and enjoyment in your coding journey!